Follow the below mentioned steps to display webp images with SwiftUI project:
First, add the SDWebImageSwiftUI package to your project following:
Second, add the SDWebImageWebPCoder package following:
Add the following code to your main file (the root file of your project) init method:
SDImageCodersManager.shared.addCoder(SDImageWebPCoder.shared)
SDWebImageDownloader.shared.setValue("image/webp,image/*,*/*;q=0.8", forHTTPHeaderField:"Accept")
The complete code of the root file will look like this:
import SwiftUI
import SDWebImage
import SDWebImageSwiftUI
import SDWebImageWebPCoder
@main
struct YourApp: App {
init() {
// WebP support
SDImageCodersManager.shared.addCoder(SDImageWebPCoder.shared)
SDWebImageDownloader.shared.setValue("image/webp,image/*,*/*;q=0.8", forHTTPHeaderField:"Accept")
}
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
You can use the following code then on your View to show the webp image:
WebImage(url: URL(string: "https://www.gstatic.com/webp/gallery/1.webp"))
.placeholder {ProgressView()}
.resizable()
.aspectRatio(contentMode: .fill)
.edgesIgnoringSafeArea(.all)
.frame(width: 400, height: 400)
.clipped()
The complete code for your View would look like this:
import SwiftUI
import SDWebImageSwiftUI
struct ContentView: View {
var body: some View {
WebImage(url: URL(string: "https://www.gstatic.com/webp/gallery/1.webp"))
.placeholder {ProgressView()}
.resizable()
.aspectRatio(contentMode: .fill)
.edgesIgnoringSafeArea(.all)
.frame(width: 400, height: 400)
.clipped()
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
The output of the above code will look like this:
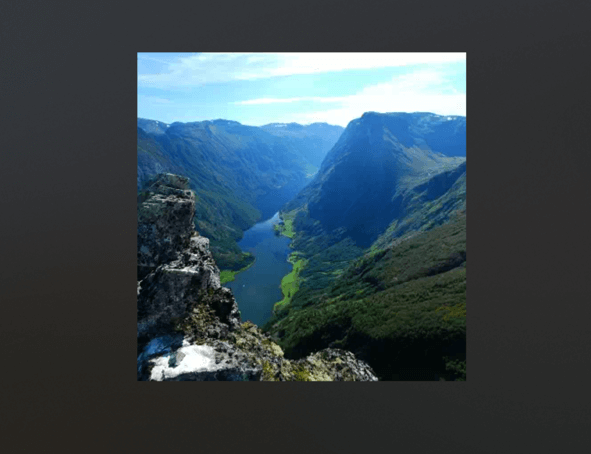