The SDWebImageSwiftUI library makes it simple to display an image’s progress by using the onProgress closure.
WebImage
Here is an example below with the code which can be used to show progress while the image is loading in SDWebImageSwiftUI WebImage:
.placeholder {ProgressView()}
The WebImage will look like this:
WebImage(url: URL(string: "https://www.gstatic.com/webp/gallery/4.webp"))
.placeholder {ProgressView()}
.resizable()
.aspectRatio(contentMode: .fill)
.edgesIgnoringSafeArea(.all)
.frame(width: 400, height: 400)
.clipped()
The complete file can be tested like this:
import SwiftUI
import SDWebImageSwiftUI
struct ContentView: View {
var body: some View {
WebImage(url: URL(string: "https://www.gstatic.com/webp/gallery/4.webp"))
.placeholder {ProgressView()}
.resizable()
.aspectRatio(contentMode: .fill)
.edgesIgnoringSafeArea(.all)
.frame(width: 400, height: 400)
.clipped()
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
The Progress will look like this while the image is loading:
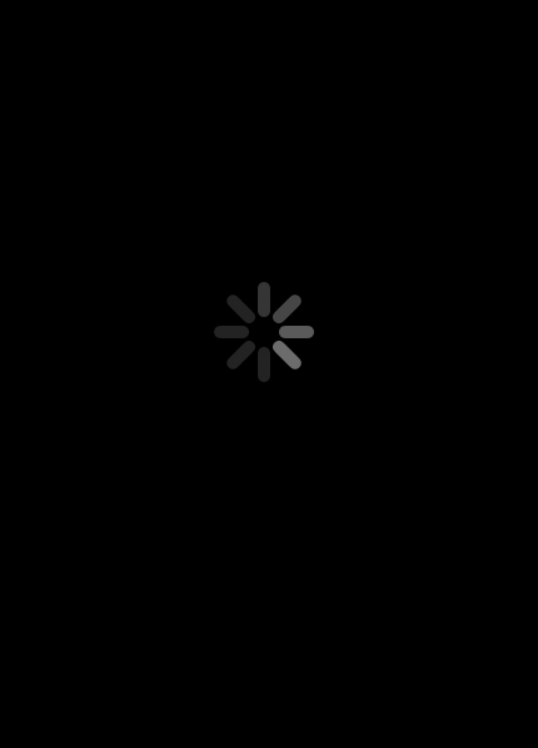
UIImage
Here’s another example of UIImage how you might use the onProgress closure to show the progress of an image download:
Image(uiImage: UIImage())
.onAppear {
let progress = Progress()
self.imageLoader.loadImage(with: url, options: [.progressiveLoad], context: &self.imageContext, progress: { receivedSize, expectedSize in
progress.completedUnitCount = Int64(receivedSize)
progress.totalUnitCount = Int64(expectedSize)
}) { (image, data, error, finished) in
if let image = image {
self.image = image
}
}
}
.onDisappear {
self.imageLoader.cancel(context: &self.imageContext)
}
This code snippet demonstrates how to load an image using the SDWebImageSwiftUI library’s loadImage function and passing a closure to the progress parameter. You can update the download progress and display it to the user inside the closure.
The Progress object is used in this example to track the download’s progress. The completedUnitCount property is updated with the number of bytes received, and the totalUnitCount property is updated with the expected number of bytes. These properties can be used to calculate and display the progress percentage to the user.
You can modify the progress display based on your specific requirements.