Below is an example to create a grid filled with colored rectangles in NavigationLink. The goal is to focus on specific NavigationLink item in the grid.
Let’s say we want to focus on the orange color navigation item link in the grid. Follow the below code to do the same:
import SwiftUI
struct ContentView: View {
var grid: [GridItem] = Array(repeating: .init(.flexible()), count: 3)
let colors: [Color] = [.red, .green, .blue, .yellow, .cyan, .orange, .gray, .indigo, .brown]
@FocusState private var focus: Color?
var body: some View {
NavigationStack {
LazyVGrid(columns: grid) {
ForEach(colors, id: \.self) { color in
NavigationLink {
// YourView()
} label: {
Rectangle()
.fill(color)
.frame(width: 200, height: 200)
}
.padding(20)
.buttonStyle(.card)
.focused($focus, equals: color)
}
}
}
.onAppear {
// Initial delay
DispatchQueue.main.asyncAfter(deadline: .now()+0.3) {
focus = .orange
}
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
With the help of the above code, the orange color navigation link item will be focused on the load of the view:
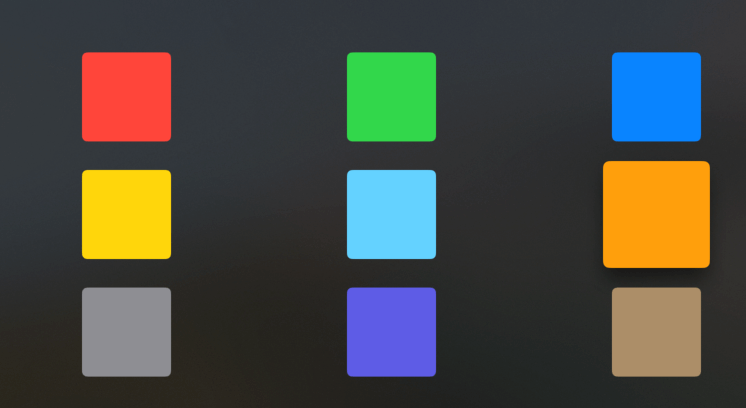
The above code creates the ContentView SwiftUI view. It has a grid of colored rectangles laid out in a lazy vertical grid layout. The grid is defined with the LazyVGrid view and is made up of an array of GridItem values that are set to be size-flexible. The colors for the rectangles are defined in the colors array.
The @FocusState property wrapper is used to track a specific color’s focus state, which is represented by the focus variable. On each rectangle, the.focused modifier is used to apply the focus state to the corresponding color. The.focused($focus, equals: colour) syntax changes the focus state of the rectangle to the color that matches the focus variable, so that when the focus variable changes, the focus state of the rectangle changes.
The onAppear modifier is used to delay the rectangle’s initial focus state to orange. It delays the focus state update by 0.3 seconds by calling the DispatchQueue.main.asyncAfter function. The NavigationStack encircles the entire view and allows you to navigate between them.
The comment “YourView()” in the NavigationLink label indicates that the developer intended to put a view inside the NavigationLink but hasn’t yet done so.
The ContentView is used to generate previews of the view in the ContentView Previews struct. To preview the view in the Xcode canvas, the previews variable is set to be an instance of ContentView.
In order to understand more about FocusState you can follow: